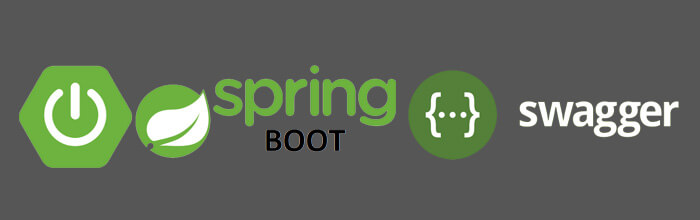
在 RESTful API 的构建中,接口文档的重要性不言而喻,而 Swagger-UI 就提供了一种自动构建接口文档的方式,它可以轻易的整合在 Spring-Boot 中,并且在写在代码中可以省去代码注释与接口文档中的重复工作,更是易于维护与更新。
- 添加依赖
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.8.0</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.8.0</version> </dependency>
- 在 Application.java 同包下创建 Swagger2 类
@Configuration @EnableSwagger2 public class Swagger2 { @Bean public Docket createRestApi() { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() //扫描路径 .apis(RequestHandlerSelectors.basePackage("com.yulaiz.dong.web")) .paths(PathSelectors.any()) .build(); } private ApiInfo apiInfo() { return new ApiInfoBuilder() .title("dong-manager") .description("董师傅的妖孽人生后台管理接口") .termsOfServiceUrl("https://dong.yulaiz.com/") .contact(new Contact("YuLaiZ", "https://yulaiz.com/", "")) .version("1.0") .build(); } }
- 添加文档内容
- @Api
添加在 Controller 上定义模块 - @ApiOperation
添加在方法上定义接口 - @ApiImplicitParams & @ApiImplicitParam
添加在方法上,用于定义方法中的 @RequestParam 参数 - @ApiModelProperty
添加在实体类的属性上,用于定义方法中的 @RequestBody 中的参数
@RestController @RequestMapping("/test") @Api(tags = "测试模块", value = "测试模块") public class Controller { @ApiOperation(value = "测试姓名", notes = "测试姓名") @ApiImplicitParams({ @ApiImplicitParam(name = "name", value = "姓名", required = true, paramType = "query"), @ApiImplicitParam(name = "age", value = "年龄", required = true, paramType = "query") }) @RequestMapping(value = "/name", method = RequestMethod.POST) public String apiParam(@RequestParam String name, @RequestParam String age) { return "YuLai"; } @ApiOperation(value = "测试", notes = "测试") @RequestMapping(value = "/info", method = RequestMethod.POST) public String apiBody(@RequestBody Info info) { return "YuLai"; } } class Info{ @ApiModelProperty("姓名") private String name; @ApiModelProperty("性别") private String sex; }
- @Api
-
启动项目,查看文档
完成上面的代码后,启动项目,访问:http://ip:port/context-path/swagger-ui.html
启动后就可以看到接口文档,并且提供了接口测试,可以直接在 Swagger-UI 进行测试
发表评论
沙发空缺中,还不快抢~